生成代码
本节正在开发中。
像ChatGPT这样的LLMs在代码生成方面非常有效。在本节中,我们将介绍许多关于如何使用ChatGPT进行代码生成的示例。
以下所有示例均使用OpenAI的Playground(聊天模式)和gpt-3.5-turbo
模型。
与所有来自OpenAI的聊天模型一样,您可以使用System Message
来定义响应的行为和格式。我们将为提示示例使用以下系统消息:
You are a helpful code assistant that can teach a junior developer how to code. Your language of choice is Python. Don't explain the code, just generate the code block itself.
基本示例
然后,User Message
将作为提示本身,包含您希望模型生成的特定代码的指令。以下是代码生成的第一个基本提示的快照。请注意,最终代码是由 Assistant
生成的。
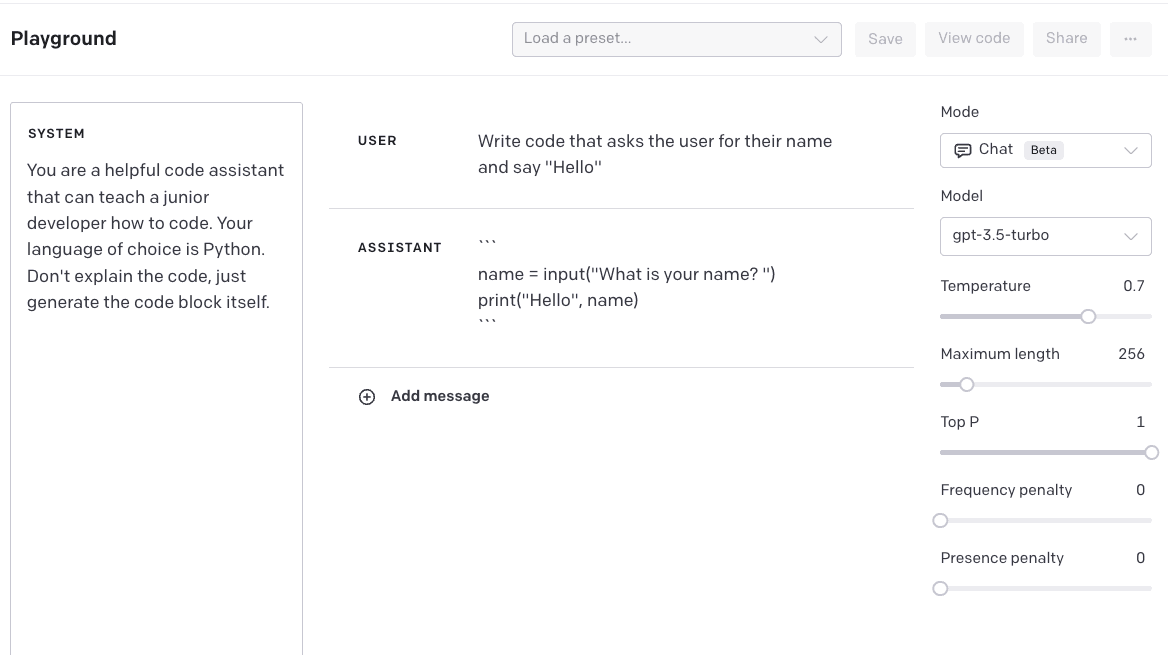
如果你想自己尝试,这里是提示:
Write code that asks the user for their name and say "Hello"
将评论转化为代码
你也可以利用这些LLM的代码生成能力,仅从注释生成代码。让我们看另一个将指令作为注释块传递的示例:
提示:
"""
1. Create a list of movies
2. Create a list of ratings for these movies
3. Combine them to make a json object of 10 movies with their ratings.
"""
输出:
movies = ["The Shawshank Redemption", "The Godfather", "The Dark Knight", "Schindler's List", "Pulp Fiction", "The Lord of the Rings: The Return of the King", "Forrest Gump", "Star Wars: Episode V - The Empire Strikes Back", "Inception", "The Silence of the Lambs"]
ratings = [9.3, 9.2, 9.0, 8.9, 8.9, 8.9, 8.8, 8.7, 8.7, 8.6]
movie_ratings = {}
for i in range(len(movies)):
movie_ratings[movies[i]] = ratings[i]
json_object = json.dumps(movie_ratings, indent=4)
print(json_object)
生成的代码看起来像是可工作的代码。然而,输出中缺少一个重要的import
语句,即import json
。虽然这些模型生成的代码可以工作,但你必须密切关注这些小细节,并始终确保测试你的代码。
完成函数或下一行
这些大型语言模型(LLMs)已经被集成到像GitHub Copilot这样的工具中,这使得它们对开发者非常有用。一个有用的功能是模型能够完成函数。
提示:
# function to multiply two numbers and add 75 to it
def multiply(
输出:
a, b):
result = a * b
result += 75
return result
MySQL 查询生成
除了上述基本的代码生成示例外,您还可以使用该模型生成有用的代码,这些代码可以用于编程的其他方面,例如创建和测试MySQL查询。
假设你有一个包含一些信息的数据集,你可以将其作为提示的一部分,然后指示它生成一个特定的查询。例如:
"""
Table departments, columns = [DepartmentId, DepartmentName]
Table students, columns = [DepartmentId, StudentId, StudentName]
Create a MySQL query for all students in the Computer Science department
"""
输出:
SELECT students.StudentId, students.StudentName
FROM students
INNER JOIN departments
ON students.DepartmentId = departments.DepartmentId
WHERE departments.DepartmentName = 'Computer Science';
模型生成了一个查询!然而,在这个例子中,我们虚构了数据库,因此我们没有数据库来测试生成的查询。如果你正在测试这个例子,创建这个数据库可能会很有用。幸运的是,你也可以使用ChatGPT来生成这个数据库。一种方法是使用上述提示的修改版本,稍微不同的指令来生成数据库模式:
Table departments, columns = [DepartmentId, DepartmentName]
Table students, columns = [DepartmentId, StudentId, StudentName]
Create a valid database schema with the above tables and columns
模型生成了以下模式:
CREATE TABLE departments (
DepartmentId INT PRIMARY KEY,
DepartmentName VARCHAR(50)
);
CREATE TABLE students (
DepartmentId INT,
StudentId INT PRIMARY KEY,
StudentName VARCHAR(50),
FOREIGN KEY (DepartmentId) REFERENCES departments(DepartmentId)
);
你可以使用类似sqliteonline.com(在新标签页中打开)的工具创建一个临时数据库。
你还需要一些虚拟数据来测试原始生成的查询是否有效。我们也可以使用ChatGPT来完成这个任务!提示可能如下所示:
CREATE TABLE departments (
DepartmentId INT PRIMARY KEY,
DepartmentName VARCHAR(50)
);
CREATE TABLE students (
DepartmentId INT,
StudentId INT PRIMARY KEY,
StudentName VARCHAR(50),
FOREIGN KEY (DepartmentId) REFERENCES departments(DepartmentId)
);
Given the database schema above, generate valid insert statements include 4 rows for each table.
带有插入语句的输出:
INSERT INTO departments (DepartmentId, DepartmentName)
VALUES (1, 'Computer Science'), (2, 'Mathematics'), (3, 'Physics'), (4, 'Biology');
INSERT INTO students (DepartmentId, StudentId, StudentName)
VALUES (1, 101, 'John Doe'), (1, 102, 'Jane Doe'), (2, 201, 'Alice Smith'), (2, 202, 'Bob Johnson');
现在你已经有了数据库和模拟数据,你可以测试原始查询。根据模拟数据,结果应该是两行,分别是Jane Doe
和John Doe
。
解释代码
如果你正在学习某种编程语言,提示模型解释某些代码片段可能会很有用。让我们重用上面生成的查询,并要求模型解释它。
如果你使用的是之前的相同系统消息,请注意你如何指示模型。例如,如果我们使用以下示例,模型会抱怨它是一个Python代码助手,但它仍然提供了解释。有时模型会拒绝生成你所指示的内容,因为它被设计为遵循系统消息。
提示:
SELECT students.StudentId, students.StudentName
FROM students
INNER JOIN departments
ON students.DepartmentId = departments.DepartmentId
WHERE departments.DepartmentName = 'Computer Science';
Explain the above SQL statement.
输出:
Sorry, as a code assistant in Python, I cannot generate SQL code. However, I can explain the purpose of the SQL statement provided.
This SQL statement selects the `StudentId` and `StudentName` columns from the `students` table, where the `DepartmentName` column in the `departments` table is equal to 'Computer Science'. To achieve this, the statement performs an inner join on the `DepartmentId` column between the `students` and `departments` tables.
In simpler terms, it is selecting the names and IDs of students who are enrolled in the Computer Science department.
编辑代码
示例即将推出!
调试代码
示例即将推出!
最佳实践
即将推出!