SAM
概述
SAM(Segment Anything Model)由Alexander Kirillov、Eric Mintun、Nikhila Ravi、Hanzi Mao、Chloe Rolland、Laura Gustafson、Tete Xiao、Spencer Whitehead、Alex Berg、Wan-Yen Lo、Piotr Dollar、Ross Girshick在Segment Anything中提出。
该模型可用于预测给定输入图像中任何感兴趣对象的分割掩码。
论文的摘要如下:
我们介绍了Segment Anything (SA)项目:一个用于图像分割的新任务、模型和数据集。通过在数据收集循环中使用我们的高效模型,我们构建了迄今为止最大的分割数据集(远远超过其他数据集),在1100万张经过许可且尊重隐私的图像上生成了超过10亿个掩码。该模型被设计和训练为可提示的,因此它可以零样本迁移到新的图像分布和任务中。我们在众多任务上评估了其能力,发现其零样本性能令人印象深刻——通常与之前完全监督的结果相当甚至更优。我们正在发布Segment Anything Model (SAM)和相应的数据集(SA-1B),包含10亿个掩码和1100万张图像,网址为https://segment-anything.com,以促进计算机视觉基础模型的研究。
提示:
- 模型预测二进制掩码,该掩码表明给定图像中是否存在感兴趣的对象。
- 如果提供了输入的2D点和/或输入的边界框,模型的预测结果会更好
- 您可以为同一张图片提示多个点,并预测一个单一的掩码。
- 目前尚不支持微调模型
- 根据论文,文本输入也应该被支持。然而,在撰写本文时,根据官方仓库,这似乎尚未得到支持。
该模型由ybelkada和ArthurZ贡献。 原始代码可以在这里找到。
以下是一个示例,展示了如何根据图像和2D点运行掩码生成:
import torch
from PIL import Image
import requests
from transformers import SamModel, SamProcessor
device = "cuda" if torch.cuda.is_available() else "cpu"
model = SamModel.from_pretrained("facebook/sam-vit-huge").to(device)
processor = SamProcessor.from_pretrained("facebook/sam-vit-huge")
img_url = "https://huggingface.co/ybelkada/segment-anything/resolve/main/assets/car.png"
raw_image = Image.open(requests.get(img_url, stream=True).raw).convert("RGB")
input_points = [[[450, 600]]] # 2D location of a window in the image
inputs = processor(raw_image, input_points=input_points, return_tensors="pt").to(device)
with torch.no_grad():
outputs = model(**inputs)
masks = processor.image_processor.post_process_masks(
outputs.pred_masks.cpu(), inputs["original_sizes"].cpu(), inputs["reshaped_input_sizes"].cpu()
)
scores = outputs.iou_scores
您还可以在处理器中处理自己的掩码,与输入图像一起传递给模型。
import torch
from PIL import Image
import requests
from transformers import SamModel, SamProcessor
device = "cuda" if torch.cuda.is_available() else "cpu"
model = SamModel.from_pretrained("facebook/sam-vit-huge").to(device)
processor = SamProcessor.from_pretrained("facebook/sam-vit-huge")
img_url = "https://huggingface.co/ybelkada/segment-anything/resolve/main/assets/car.png"
raw_image = Image.open(requests.get(img_url, stream=True).raw).convert("RGB")
mask_url = "https://huggingface.co/ybelkada/segment-anything/resolve/main/assets/car.png"
segmentation_map = Image.open(requests.get(mask_url, stream=True).raw).convert("1")
input_points = [[[450, 600]]] # 2D location of a window in the image
inputs = processor(raw_image, input_points=input_points, segmentation_maps=segmentation_map, return_tensors="pt").to(device)
with torch.no_grad():
outputs = model(**inputs)
masks = processor.image_processor.post_process_masks(
outputs.pred_masks.cpu(), inputs["original_sizes"].cpu(), inputs["reshaped_input_sizes"].cpu()
)
scores = outputs.iou_scores
资源
一份官方的Hugging Face和社区(由🌎表示)资源列表,帮助您开始使用SAM。
- Demo notebook 用于使用模型。
- Demo notebook 用于使用自动掩码生成管道。
- Demo notebook 用于使用MedSAM进行推理,这是SAM在医学领域的微调版本。🌎
- Demo notebook 用于在自定义数据上微调模型。🌎
SlimSAM
SlimSAM,SAM的剪枝版本,由Zigeng Chen等人在0.1% Data Makes Segment Anything Slim中提出。SlimSAM在保持相同性能的同时,显著减小了SAM模型的尺寸。
检查点可以在hub上找到,它们可以作为SAM的直接替代品使用。
Grounded SAM
可以将Grounding DINO与SAM结合,用于基于文本的掩码生成,如Grounded SAM: Assembling Open-World Models for Diverse Visual Tasks中介绍的那样。您可以参考这个演示笔记本 🌍 获取详细信息。
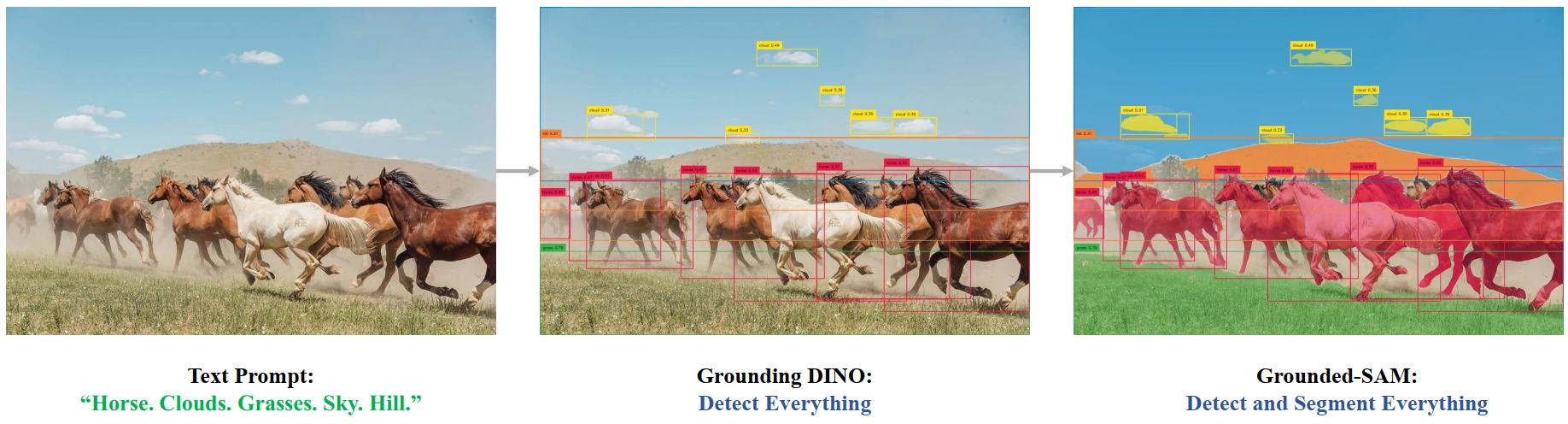
SamConfig
类 transformers.SamConfig
< source >( vision_config = 无 prompt_encoder_config = 无 mask_decoder_config = 无 initializer_range = 0.02 **kwargs )
参数
- vision_config (Union[
dict
,SamVisionConfig
], optional) — 用于初始化SamVisionConfig的配置选项字典。 - prompt_encoder_config (Union[
dict
,SamPromptEncoderConfig
], optional) — 用于初始化SamPromptEncoderConfig的配置选项字典。 - mask_decoder_config (Union[
dict
,SamMaskDecoderConfig
], optional) — 用于初始化SamMaskDecoderConfig的配置选项字典。 - kwargs (可选) — 关键字参数字典。
SamConfig 是用于存储 SamModel 配置的配置类。它用于根据指定的参数实例化一个 SAM 模型,定义视觉模型、提示编码器模型和掩码解码器配置。使用默认值实例化配置将产生与 facebook/sam-vit-huge 架构类似的配置。
配置对象继承自PretrainedConfig,可用于控制模型输出。阅读PretrainedConfig的文档以获取更多信息。
示例:
>>> from transformers import (
... SamVisionConfig,
... SamPromptEncoderConfig,
... SamMaskDecoderConfig,
... SamModel,
... )
>>> # Initializing a SamConfig with `"facebook/sam-vit-huge"` style configuration
>>> configuration = SamConfig()
>>> # Initializing a SamModel (with random weights) from the `"facebook/sam-vit-huge"` style configuration
>>> model = SamModel(configuration)
>>> # Accessing the model configuration
>>> configuration = model.config
>>> # We can also initialize a SamConfig from a SamVisionConfig, SamPromptEncoderConfig, and SamMaskDecoderConfig
>>> # Initializing SAM vision, SAM Q-Former and language model configurations
>>> vision_config = SamVisionConfig()
>>> prompt_encoder_config = SamPromptEncoderConfig()
>>> mask_decoder_config = SamMaskDecoderConfig()
>>> config = SamConfig(vision_config, prompt_encoder_config, mask_decoder_config)
SamVisionConfig
类 transformers.SamVisionConfig
< source >( hidden_size = 768 output_channels = 256 num_hidden_layers = 12 num_attention_heads = 12 num_channels = 3 image_size = 1024 patch_size = 16 hidden_act = 'gelu' layer_norm_eps = 1e-06 attention_dropout = 0.0 initializer_range = 1e-10 qkv_bias = True mlp_ratio = 4.0 use_abs_pos = True use_rel_pos = True window_size = 14 global_attn_indexes = [2, 5, 8, 11] num_pos_feats = 128 mlp_dim = None **kwargs )
参数
- hidden_size (
int
, optional, 默认为 768) — 编码器层和池化层的维度。 - output_channels (
int
, optional, 默认为 256) — Patch Encoder 中输出通道的维度。 - num_hidden_layers (
int
, 可选, 默认为 12) — Transformer 编码器中的隐藏层数量。 - num_attention_heads (
int
, optional, 默认为 12) — Transformer 编码器中每个注意力层的注意力头数。 - num_channels (
int
, optional, defaults to 3) — 输入图像中的通道数。 - image_size (
int
, optional, 默认为 1024) — 预期分辨率。调整后的输入图像的目标大小。 - patch_size (
int
, optional, defaults to 16) — 从输入图像中提取的补丁的大小。 - hidden_act (
str
, optional, defaults to"gelu"
) — 非线性激活函数(函数或字符串) - layer_norm_eps (
float
, optional, defaults to 1e-06) — 层归一化层使用的epsilon值。 - attention_dropout (
float
, optional, 默认为 0.0) — 注意力概率的丢弃比例。 - initializer_range (
float
, optional, 默认为 1e-10) — 用于初始化所有权重矩阵的 truncated_normal_initializer 的标准差。 - qkv_bias (
bool
, optional, defaults toTrue
) — 是否在查询、键、值投影中添加偏置。 - mlp_ratio (
float
, optional, defaults to 4.0) — MLP隐藏维度与嵌入维度的比率。 - use_abs_pos (
bool
, optional, defaults toTrue
) — 是否使用绝对位置嵌入。 - use_rel_pos (
bool
, optional, defaults toTrue
) — 是否使用相对位置嵌入。 - window_size (
int
, optional, defaults to 14) — 相对位置的窗口大小。 - global_attn_indexes (
List[int]
, 可选, 默认为[2, 5, 8, 11]
) — 全局注意力层的索引。 - num_pos_feats (
int
, optional, defaults to 128) — 位置嵌入的维度。 - mlp_dim (
int
, optional) — Transformer编码器中MLP层的维度。如果为None
,则默认为mlp_ratio * hidden_size
.
这是用于存储SamVisionModel
配置的配置类。它用于根据指定的参数实例化SAM视觉编码器,定义模型架构。实例化配置默认值将产生与SAM ViT-h facebook/sam-vit-huge架构类似的配置。
配置对象继承自PretrainedConfig,可用于控制模型输出。阅读PretrainedConfig的文档以获取更多信息。
SamMaskDecoderConfig
类 transformers.SamMaskDecoderConfig
< source >( hidden_size = 256 hidden_act = 'relu' mlp_dim = 2048 num_hidden_layers = 2 num_attention_heads = 8 attention_downsample_rate = 2 num_multimask_outputs = 3 iou_head_depth = 3 iou_head_hidden_dim = 256 layer_norm_eps = 1e-06 **kwargs )
参数
- hidden_size (
int
, optional, defaults to 256) — 隐藏状态的维度。 - hidden_act (
str
, optional, defaults to"relu"
) — 在SamMaskDecoder
模块中使用的非线性激活函数。 - mlp_dim (
int
, optional, 默认为 2048) — Transformer 编码器中“中间”(即前馈)层的维度。 - num_hidden_layers (
int
, optional, defaults to 2) — Transformer编码器中的隐藏层数量。 - num_attention_heads (
int
, optional, defaults to 8) — Transformer编码器中每个注意力层的注意力头数。 - attention_downsample_rate (
int
, optional, defaults to 2) — 注意力层的下采样率。 - num_multimask_outputs (
int
, 可选, 默认为 3) —SamMaskDecoder
模块的输出数量。在 Segment Anything 论文中,此值设置为 3。 - iou_head_depth (
int
, optional, 默认为 3) — IoU 头模块中的层数。 - iou_head_hidden_dim (
int
, optional, defaults to 256) — IoU头模块中隐藏状态的维度。 - layer_norm_eps (
float
, optional, defaults to 1e-06) — 层归一化层使用的epsilon值。
这是用于存储SamMaskDecoder
配置的配置类。它用于根据指定的参数实例化一个SAM掩码解码器,定义模型架构。实例化配置默认值将产生与SAM-vit-h facebook/sam-vit-huge架构类似的配置。
配置对象继承自PretrainedConfig,可用于控制模型输出。阅读PretrainedConfig的文档以获取更多信息。
SamPromptEncoderConfig
类 transformers.SamPromptEncoderConfig
< source >( hidden_size = 256 image_size = 1024 patch_size = 16 mask_input_channels = 16 num_point_embeddings = 4 hidden_act = 'gelu' layer_norm_eps = 1e-06 **kwargs )
参数
- hidden_size (
int
, optional, defaults to 256) — 隐藏状态的维度。 - image_size (
int
, optional, 默认为 1024) — 图像的预期输出分辨率。 - patch_size (
int
, optional, defaults to 16) — 每个补丁的大小(分辨率)。 - mask_input_channels (
int
, 可选, 默认为 16) — 输入到MaskDecoder
模块的通道数。 - num_point_embeddings (
int
, optional, defaults to 4) — 要使用的点嵌入数量。 - hidden_act (
str
, optional, defaults to"gelu"
) — 编码器和池化器中的非线性激活函数。
这是用于存储SamPromptEncoder
配置的配置类。SamPromptEncoder
模块用于编码输入的2D点和边界框。实例化配置默认值将产生与SAM-vit-h facebook/sam-vit-huge架构类似的配置。
配置对象继承自PretrainedConfig,可用于控制模型输出。阅读PretrainedConfig的文档以获取更多信息。
SamProcessor
类 transformers.SamProcessor
< source >( image_processor )
参数
- image_processor (
SamImageProcessor
) — 一个 SamImageProcessor 的实例。图像处理器是一个必需的输入。
构建一个SAM处理器,它将一个SAM图像处理器和一个2D点及边界框处理器封装成一个单一的处理器。
SamProcessor 提供了 SamImageProcessor 的所有功能。更多信息请参阅 call() 的文档字符串。
SamImageProcessor
类 transformers.SamImageProcessor
< source >( do_resize: bool = True size: typing.Dict[str, int] = None mask_size: typing.Dict[str, int] = None resample: Resampling =
参数
- do_resize (
bool
, 可选, 默认为True
) — 是否将图像的(高度,宽度)尺寸调整为指定的size
。可以在preprocess
方法中通过do_resize
参数覆盖此设置。 - size (
dict
, optional, defaults to{"longest_edge" -- 1024}
): 调整后输出图像的大小。将图像的最长边调整为匹配size["longest_edge"]
,同时保持宽高比。可以在preprocess
方法中通过size
参数覆盖此设置。 - mask_size (
dict
, 可选, 默认为{"longest_edge" -- 256}
): 调整大小后输出分割图的大小。将图像的最长边调整为匹配size["longest_edge"]
同时保持宽高比。可以在preprocess
方法中通过mask_size
参数 覆盖此设置。 - resample (
PILImageResampling
, 可选, 默认为Resampling.BILINEAR
) — 如果调整图像大小,则使用的重采样过滤器。可以在preprocess
方法中通过resample
参数覆盖。 - do_rescale (
bool
, 可选, 默认为True
) — 是否通过指定的比例rescale_factor
来重新缩放图像。可以在preprocess
方法中通过do_rescale
参数进行覆盖。 - rescale_factor (
int
或float
, 可选, 默认为1/255
) — 如果重新缩放图像,则使用的缩放因子。仅在do_rescale
设置为True
时有效。可以被preprocess
方法中的rescale_factor
参数覆盖。 - do_normalize (
bool
, 可选, 默认为True
) — 是否对图像进行归一化。可以在preprocess
方法中通过do_normalize
参数进行覆盖。可以在preprocess
方法中通过do_normalize
参数进行覆盖。 - image_mean (
float
或List[float]
, 可选, 默认为IMAGENET_DEFAULT_MEAN
) — 如果对图像进行归一化,则使用的均值。这是一个浮点数或与图像通道数长度相同的浮点数列表。可以在preprocess
方法中通过image_mean
参数进行覆盖。可以在preprocess
方法中通过image_mean
参数进行覆盖。 - image_std (
float
或List[float]
, 可选, 默认为IMAGENET_DEFAULT_STD
) — 如果对图像进行归一化,则使用的标准差。这是一个浮点数或与图像通道数长度相同的浮点数列表。可以在preprocess
方法中通过image_std
参数覆盖。 可以在preprocess
方法中通过image_std
参数覆盖。 - do_pad (
bool
, 可选, 默认为True
) — 是否将图像填充到指定的pad_size
。可以在preprocess
方法中通过do_pad
参数覆盖此设置。 - pad_size (
dict
, 可选, 默认为{"height" -- 1024, "width": 1024}
): 填充后输出图像的大小。可以通过preprocess
方法中的pad_size
参数进行覆盖。 - mask_pad_size (
dict
, 可选, 默认为{"height" -- 256, "width": 256}
): 填充后输出分割图的大小。可以通过preprocess
方法中的mask_pad_size
参数进行覆盖。 - do_convert_rgb (
bool
, optional, defaults toTrue
) — 是否将图像转换为RGB。
构建一个SAM图像处理器。
filter_masks
< source >( masks iou_scores original_size cropped_box_image pred_iou_thresh = 0.88 stability_score_thresh = 0.95 mask_threshold = 0 stability_score_offset = 1 return_tensors = 'pt' )
参数
- masks (
Union[torch.Tensor, tf.Tensor]
) — 输入掩码. - iou_scores (
Union[torch.Tensor, tf.Tensor]
) — IoU 分数列表. - original_size (
Tuple[int,int]
) — 原始图像的大小。 - cropped_box_image (
np.array
) — 裁剪后的图像. - pred_iou_thresh (
float
, optional, defaults to 0.88) — iou分数的阈值。 - stability_score_thresh (
float
, optional, defaults to 0.95) — 稳定性分数的阈值。 - mask_threshold (
float
, optional, defaults to 0) — 预测掩码的阈值。 - stability_score_offset (
float
, 可选, 默认为 1) — 用于_compute_stability_score
方法中的稳定性分数偏移量。 - return_tensors (
str
, 可选, 默认为pt
) — 如果为pt
,返回torch.Tensor
。如果为tf
,返回tf.Tensor
.
通过选择满足多个条件的预测掩码进行过滤。第一个条件是iou分数需要大于pred_iou_thresh
。第二个条件是稳定性分数需要大于stability_score_thresh
。该方法还将预测的掩码转换为边界框,并在必要时填充预测的掩码。
generate_crop_boxes
< source >( image target_size crop_n_layers: int = 0 overlap_ratio: float = 0.3413333333333333 points_per_crop: typing.Optional[int] = 32 crop_n_points_downscale_factor: typing.Optional[typing.List[int]] = 1 device: typing.Optional[ForwardRef('torch.device')] = None input_data_format: typing.Union[str, transformers.image_utils.ChannelDimension, NoneType] = None return_tensors: str = 'pt' )
参数
- image (
np.array
) — 输入原始图像 - target_size (
int
) — 调整后图像的目标大小 - crop_n_layers (
int
, 可选, 默认为 0) — 如果大于0,将在图像的裁剪区域上再次运行掩码预测。设置运行的层数,其中 每层有 2**i_layer 数量的图像裁剪区域。 - overlap_ratio (
float
, optional, 默认为 512/1500) — 设置裁剪重叠的程度。在第一层裁剪中,裁剪将重叠图像长度的这个比例。后续层中更多的裁剪会缩小这个重叠比例。 - points_per_crop (
int
, optional, defaults to 32) — 从每个作物中采样的点数。 - crop_n_points_downscale_factor (
List[int]
, 可选, 默认为 1) — 在第n层中,每边采样的点数按crop_n_points_downscale_factor**n的比例缩小。 - device (
torch.device
, optional, 默认为 None) — 用于计算的设备。如果为 None,将使用 cpu。 - input_data_format (
str
或ChannelDimension
, 可选) — 输入图像的通道维度格式。如果未提供,将自动推断。 - return_tensors (
str
, 可选, 默认为pt
) — 如果为pt
,返回torch.Tensor
。如果为tf
,返回tf.Tensor
.
生成不同大小的裁剪框列表。每一层有 (2i)2 个框,其中 i 表示层数。
pad_image
< source >( image: ndarray pad_size: typing.Dict[str, int] data_format: typing.Union[str, transformers.image_utils.ChannelDimension, NoneType] = None input_data_format: typing.Union[str, transformers.image_utils.ChannelDimension, NoneType] = None **kwargs )
将图像填充到(pad_size["height"], pad_size["width"])
,并在右侧和底部用零填充。
post_process_for_mask_generation
< source >( all_masks all_scores all_boxes crops_nms_thresh return_tensors = 'pt' )
参数
- all_masks (
Union[List[torch.Tensor], List[tf.Tensor]]
) — 所有预测的分割掩码列表 - all_scores (
Union[List[torch.Tensor], List[tf.Tensor]]
) — 所有预测的iou分数列表 - all_boxes (
Union[List[torch.Tensor], List[tf.Tensor]]
) — 预测掩码的所有边界框列表 - crops_nms_thresh (
float
) — NMS(非极大值抑制)算法的阈值。 - return_tensors (
str
, 可选, 默认为pt
) — 如果为pt
,返回torch.Tensor
。如果为tf
,返回tf.Tensor
.
对通过调用非最大抑制算法生成的预测掩码进行后处理。
post_process_masks
< source >( masks original_sizes reshaped_input_sizes mask_threshold = 0.0 binarize = True pad_size = None return_tensors = 'pt' ) → (Union[torch.Tensor, tf.Tensor]
)
参数
- masks (
Union[List[torch.Tensor], List[np.ndarray], List[tf.Tensor]]
) — 来自mask_decoder的批量掩码,格式为(batch_size, num_channels, height, width)。 - original_sizes (
Union[torch.Tensor, tf.Tensor, List[Tuple[int,int]]]
) — 每张图像在调整大小到模型预期输入形状之前的原始尺寸,格式为(高度,宽度)。 - reshaped_input_sizes (
Union[torch.Tensor, tf.Tensor, List[Tuple[int,int]]]
) — 每个图像输入模型时的大小,格式为(高度,宽度)。用于去除填充。 - mask_threshold (
float
, optional, defaults to 0.0) — 用于二值化掩码的阈值。 - binarize (
bool
, optional, defaults toTrue
) — 是否对掩码进行二值化处理。 - pad_size (
int
, 可选, 默认为self.pad_size
) — 图像在传递给模型之前被填充到的目标大小。如果为None,则假定目标大小为处理器的pad_size
. - return_tensors (
str
, 可选, 默认为"pt"
) — 如果为"pt"
,返回 PyTorch 张量。如果为"tf"
,返回 TensorFlow 张量。
返回
(Union[torch.Tensor, tf.Tensor]
)
批量掩码的格式为 batch_size, num_channels, height, width),其中 (height, width) 由 original_size 给出。
移除填充并将掩码放大到原始图像大小。
预处理
< source >( images: typing.Union[ForwardRef('PIL.Image.Image'), numpy.ndarray, ForwardRef('torch.Tensor'), typing.List[ForwardRef('PIL.Image.Image')], typing.List[numpy.ndarray], typing.List[ForwardRef('torch.Tensor')]] segmentation_maps: typing.Union[ForwardRef('PIL.Image.Image'), numpy.ndarray, ForwardRef('torch.Tensor'), typing.List[ForwardRef('PIL.Image.Image')], typing.List[numpy.ndarray], typing.List[ForwardRef('torch.Tensor')], NoneType] = None do_resize: typing.Optional[bool] = None size: typing.Optional[typing.Dict[str, int]] = None mask_size: typing.Optional[typing.Dict[str, int]] = None resample: typing.Optional[ForwardRef('PILImageResampling')] = None do_rescale: typing.Optional[bool] = None rescale_factor: typing.Union[int, float, NoneType] = None do_normalize: typing.Optional[bool] = None image_mean: typing.Union[float, typing.List[float], NoneType] = None image_std: typing.Union[float, typing.List[float], NoneType] = None do_pad: typing.Optional[bool] = None pad_size: typing.Optional[typing.Dict[str, int]] = None mask_pad_size: typing.Optional[typing.Dict[str, int]] = None do_convert_rgb: typing.Optional[bool] = None return_tensors: typing.Union[str, transformers.utils.generic.TensorType, NoneType] = None data_format: ChannelDimension =
参数
- 图像 (
ImageInput
) — 要预处理的图像。期望输入单个或批量的图像,像素值范围在0到255之间。如果传入的图像像素值在0到1之间,请设置do_rescale=False
. - segmentation_maps (
ImageInput
, optional) — 用于预处理的图像分割图. - do_resize (
bool
, optional, defaults toself.do_resize
) — 是否调整图像大小. - size (
Dict[str, int]
, 可选, 默认为self.size
) — 控制图像在resize
之后的大小。图像的最长边将调整为size["longest_edge"]
,同时保持宽高比。 - mask_size (
Dict[str, int]
, 可选, 默认为self.mask_size
) — 控制resize
后分割图的大小。图像的最长边将被调整为size["longest_edge"]
,同时保持宽高比。 - resample (
PILImageResampling
, 可选, 默认为self.resample
) —PILImageResampling
过滤器用于调整图像大小时使用,例如PILImageResampling.BILINEAR
. - do_rescale (
bool
, optional, defaults toself.do_rescale
) — 是否通过缩放因子重新缩放图像像素值。 - rescale_factor (
int
orfloat
, optional, defaults toself.rescale_factor
) — 应用于图像像素值的重新缩放因子。 - do_normalize (
bool
, optional, defaults toself.do_normalize
) — 是否对图像进行归一化处理。 - image_mean (
float
或List[float]
, 可选, 默认为self.image_mean
) — 如果do_normalize
设置为True
,则用于归一化图像的图像均值. - image_std (
float
或List[float]
, 可选, 默认为self.image_std
) — 如果do_normalize
设置为True
,则用于归一化图像的标准差。 - do_pad (
bool
, 可选, 默认为self.do_pad
) — 是否对图像进行填充. - pad_size (
Dict[str, int]
, 可选, 默认为self.pad_size
) — 控制应用于图像的填充大小。如果do_pad
设置为True
,则图像将填充到pad_size["height"]
和pad_size["width"]
。 - mask_pad_size (
Dict[str, int]
, optional, defaults toself.mask_pad_size
) — 控制应用于分割图的填充大小。如果do_pad
设置为True
,图像将被填充到mask_pad_size["height"]
和mask_pad_size["width"]
。 - do_convert_rgb (
bool
, optional, defaults toself.do_convert_rgb
) — 是否将图像转换为RGB. - return_tensors (
str
或TensorType
, 可选) — 返回的张量类型。可以是以下之一:- 未设置:返回一个
np.ndarray
列表。 TensorType.TENSORFLOW
或'tf'
:返回一个类型为tf.Tensor
的批次。TensorType.PYTORCH
或'pt'
:返回一个类型为torch.Tensor
的批次。TensorType.NUMPY
或'np'
:返回一个类型为np.ndarray
的批次。TensorType.JAX
或'jax'
:返回一个类型为jax.numpy.ndarray
的批次。
- 未设置:返回一个
- data_format (
ChannelDimension
或str
, 可选, 默认为ChannelDimension.FIRST
) — 输出图像的通道维度格式。可以是以下之一:"channels_first"
或ChannelDimension.FIRST
: 图像格式为 (num_channels, height, width)。"channels_last"
或ChannelDimension.LAST
: 图像格式为 (height, width, num_channels)。- 未设置:使用输入图像的通道维度格式。
- input_data_format (
ChannelDimension
或str
, 可选) — 输入图像的通道维度格式。如果未设置,则从输入图像推断通道维度格式。可以是以下之一:"channels_first"
或ChannelDimension.FIRST
: 图像格式为 (num_channels, height, width)。"channels_last"
或ChannelDimension.LAST
: 图像格式为 (height, width, num_channels)。"none"
或ChannelDimension.NONE
: 图像格式为 (height, width)。
预处理一张图像或一批图像。
调整大小
< source >( image: ndarray size: typing.Dict[str, int] resample: Resampling = np.ndarray
参数
- image (
np.ndarray
) — 要调整大小的图像。 - size (
Dict[str, int]
) — 指定输出图像大小的字典,格式为{"longest_edge": int}
。图像的最长边将调整为指定大小,而另一边将调整以保持宽高比。 - resample —
PILImageResampling
过滤器用于调整图像大小时使用,例如PILImageResampling.BILINEAR
. - data_format (
ChannelDimension
或str
, 可选) — 输出图像的通道维度格式。如果未设置,则使用输入图像的通道维度格式。可以是以下之一:"channels_first"
或ChannelDimension.FIRST
: 图像格式为 (num_channels, height, width)。"channels_last"
或ChannelDimension.LAST
: 图像格式为 (height, width, num_channels)。
- input_data_format (
ChannelDimension
或str
, 可选) — 输入图像的通道维度格式。如果未设置,则从输入图像推断通道维度格式。可以是以下之一:"channels_first"
或ChannelDimension.FIRST
: 图像格式为 (num_channels, height, width)。"channels_last"
或ChannelDimension.LAST
: 图像格式为 (height, width, num_channels)。
返回
np.ndarray
调整大小后的图像。
调整图像大小为 (size["height"], size["width"])
。
SamModel
类 transformers.SamModel
< source >( config )
参数
- config (SamConfig) — 包含模型所有参数的模型配置类。 使用配置文件初始化不会加载与模型相关的权重,仅加载配置。查看 from_pretrained() 方法以加载模型权重。
Segment Anything Model (SAM) 用于生成分割掩码,给定输入图像和可选的2D位置和边界框。 该模型继承自 PreTrainedModel。请查看超类文档以了解库为其所有模型实现的通用方法(如下载或保存、调整输入嵌入的大小、修剪头部等)。
该模型也是一个PyTorch torch.nn.Module 子类。 将其作为常规的PyTorch模块使用,并参考PyTorch文档以获取与一般使用和行为相关的所有信息。
前进
< source >( pixel_values: typing.Optional[torch.FloatTensor] = None input_points: typing.Optional[torch.FloatTensor] = None input_labels: typing.Optional[torch.LongTensor] = None input_boxes: typing.Optional[torch.FloatTensor] = None input_masks: typing.Optional[torch.LongTensor] = None image_embeddings: typing.Optional[torch.FloatTensor] = None multimask_output: bool = True attention_similarity: typing.Optional[torch.FloatTensor] = None target_embedding: typing.Optional[torch.FloatTensor] = None output_attentions: typing.Optional[bool] = None output_hidden_states: typing.Optional[bool] = None return_dict: typing.Optional[bool] = None **kwargs )
参数
- pixel_values (
torch.FloatTensor
of shape(batch_size, num_channels, height, width)
) — 像素值。像素值可以使用SamProcessor获取。详情请参见SamProcessor.__call__()
。 - input_points (
torch.FloatTensor
of shape(batch_size, num_points, 2)
) — Input 2D spatial points, this is used by the prompt encoder to encode the prompt. Generally yields to much better results. The points can be obtained by passing a list of list of list to the processor that will create correspondingtorch
tensors of dimension 4. The first dimension is the image batch size, the second dimension is the point batch size (i.e. how many segmentation masks do we want the model to predict per input point), the third dimension is the number of points per segmentation mask (it is possible to pass multiple points for a single mask), and the last dimension is the x (vertical) and y (horizontal) coordinates of the point. If a different number of points is passed either for each image, or for each mask, the processor will create “PAD” points that will correspond to the (0, 0) coordinate, and the computation of the embedding will be skipped for these points using the labels. - input_labels (
torch.LongTensor
of shape(batch_size, point_batch_size, num_points)
) — Input labels for the points, this is used by the prompt encoder to encode the prompt. According to the official implementation, there are 3 types of labels1
: the point is a point that contains the object of interest0
: the point is a point that does not contain the object of interest-1
: the point corresponds to the background
我们添加了标签:
-10
: the point is a padding point, thus should be ignored by the prompt encoder
填充标签应该由处理器自动完成。
- input_boxes (
torch.FloatTensor
of shape(batch_size, num_boxes, 4)
) — Input boxes for the points, this is used by the prompt encoder to encode the prompt. Generally yields to much better generated masks. The boxes can be obtained by passing a list of list of list to the processor, that will generate atorch
tensor, with each dimension corresponding respectively to the image batch size, the number of boxes per image and the coordinates of the top left and botton right point of the box. In the order (x1
,y1
,x2
,y2
):x1
: the x coordinate of the top left point of the input boxy1
: the y coordinate of the top left point of the input boxx2
: the x coordinate of the bottom right point of the input boxy2
: the y coordinate of the bottom right point of the input box
- input_masks (
torch.FloatTensor
of shape(batch_size, image_size, image_size)
) — SAM 模型也接受分割掩码作为输入。掩码将通过提示编码器嵌入以生成相应的嵌入,该嵌入随后将输入到掩码解码器。这些掩码需要用户手动输入,并且它们的形状需要为 (batch_size
,image_size
,image_size
)。 - image_embeddings (
torch.FloatTensor
of shape(batch_size, output_channels, window_size, window_size)
) — 图像嵌入,这被掩码解码器用来生成掩码和iou分数。为了更高效的内存计算,用户可以首先使用get_image_embeddings
方法检索图像嵌入,然后将它们输入到forward
方法中,而不是输入pixel_values
。 - multimask_output (
bool
, optional) — 在原始实现和论文中,模型总是为每张图像(或每个点/每个边界框,如果相关)输出3个掩码。然而,通过指定multimask_output=False
,可以只输出一个掩码,该掩码对应于“最佳”掩码。 - attention_similarity (
torch.FloatTensor
, 可选) — 注意力相似性张量,用于在模型用于个性化时提供给掩码解码器以进行目标引导的注意力,如PerSAM中介绍的。 - target_embedding (
torch.FloatTensor
, 可选) — 目标概念的嵌入,用于在模型用于个性化时提供给掩码解码器进行目标语义提示,如PerSAM中介绍的那样。 - output_attentions (
bool
, 可选) — 是否返回所有注意力层的注意力张量。有关更多详细信息,请参见返回张量下的attentions
。 - output_hidden_states (
bool
, 可选) — 是否返回所有层的隐藏状态。有关更多详细信息,请参见返回张量下的hidden_states
。 - return_dict (
bool
, optional) — 是否返回一个ModelOutput而不是一个普通的元组。 - 示例 —
- ```python —
从PIL导入图像 导入请求 从transformers导入自动模型, 自动处理器
SamModel 的前向方法,重写了 __call__
特殊方法。
尽管前向传递的配方需要在此函数内定义,但之后应该调用Module
实例而不是这个,因为前者负责运行预处理和后处理步骤,而后者会默默地忽略它们。
TFSamModel
类 transformers.TFSamModel
< source >( config **kwargs )
参数
- config (SamConfig) — 包含模型所有参数的模型配置类。 使用配置文件初始化不会加载与模型相关的权重,只会加载配置。查看 from_pretrained() 方法以加载模型权重。
Segment Anything Model (SAM) 用于生成分割掩码,给定输入图像和可选的2D位置和边界框。 该模型继承自 TFPreTrainedModel。请查看超类文档以了解库为其所有模型实现的通用方法(如下载或保存、调整输入嵌入的大小、修剪头部等)。
该模型也是一个 TensorFlow keras.Model 子类。可以将其作为常规的 TensorFlow 模型使用,并参考 TensorFlow 文档以了解与一般使用和行为相关的所有事项。
调用
< source >( pixel_values: TFModelInputType | None = None input_points: tf.Tensor | None = None input_labels: tf.Tensor | None = None input_boxes: tf.Tensor | None = None input_masks: tf.Tensor | None = None image_embeddings: tf.Tensor | None = None multimask_output: bool = True output_attentions: bool | None = None output_hidden_states: bool | None = None return_dict: bool | None = None training: bool = False **kwargs )
参数
- pixel_values (
tf.Tensor
of shape(batch_size, num_channels, height, width)
) — 像素值。像素值可以使用SamProcessor获取。详情请参见SamProcessor.__call__()
。 - input_points (
tf.Tensor
of shape(batch_size, num_points, 2)
) — Input 2D spatial points, this is used by the prompt encoder to encode the prompt. Generally yields to much better results. The points can be obtained by passing a list of list of list to the processor that will create correspondingtf
tensors of dimension 4. The first dimension is the image batch size, the second dimension is the point batch size (i.e. how many segmentation masks do we want the model to predict per input point), the third dimension is the number of points per segmentation mask (it is possible to pass multiple points for a single mask), and the last dimension is the x (vertical) and y (horizontal) coordinates of the point. If a different number of points is passed either for each image, or for each mask, the processor will create “PAD” points that will correspond to the (0, 0) coordinate, and the computation of the embedding will be skipped for these points using the labels. - input_labels (
tf.Tensor
of shape(batch_size, point_batch_size, num_points)
) — Input labels for the points, this is used by the prompt encoder to encode the prompt. According to the official implementation, there are 3 types of labels1
: the point is a point that contains the object of interest0
: the point is a point that does not contain the object of interest-1
: the point corresponds to the background
我们添加了标签:
-10
: the point is a padding point, thus should be ignored by the prompt encoder
填充标签应该由处理器自动完成。
- input_boxes (
tf.Tensor
of shape(batch_size, num_boxes, 4)
) — Input boxes for the points, this is used by the prompt encoder to encode the prompt. Generally yields to much better generated masks. The boxes can be obtained by passing a list of list of list to the processor, that will generate atf
tensor, with each dimension corresponding respectively to the image batch size, the number of boxes per image and the coordinates of the top left and botton right point of the box. In the order (x1
,y1
,x2
,y2
):x1
: the x coordinate of the top left point of the input boxy1
: the y coordinate of the top left point of the input boxx2
: the x coordinate of the bottom right point of the input boxy2
: the y coordinate of the bottom right point of the input box
- input_masks (
tf.Tensor
of shape(batch_size, image_size, image_size)
) — SAM 模型也接受分割掩码作为输入。掩码将通过提示编码器嵌入,以生成相应的嵌入,该嵌入稍后将输入到掩码解码器。这些掩码需要用户手动输入,并且它们的形状需要为 (batch_size
,image_size
,image_size
)。 - image_embeddings (
tf.Tensor
of shape(batch_size, output_channels, window_size, window_size)
) — 图像嵌入,这被掩码解码器用来生成掩码和iou分数。为了更高效的内存计算,用户可以首先使用get_image_embeddings
方法检索图像嵌入,然后将它们提供给call
方法,而不是提供pixel_values
。 - multimask_output (
bool
, optional) — 在原始实现和论文中,模型总是为每张图像(或每个点/每个边界框,如果相关)输出3个掩码。然而,通过指定multimask_output=False
,可以只输出一个掩码,该掩码对应于“最佳”掩码。 - output_attentions (
bool
, 可选) — 是否返回所有注意力层的注意力张量。有关更多详细信息,请参见返回张量中的attentions
。 - output_hidden_states (
bool
, 可选) — 是否返回所有层的隐藏状态。有关更多详细信息,请参见返回张量下的hidden_states
。 - return_dict (
bool
, 可选) — 是否返回一个ModelOutput而不是一个普通的元组。
TFSamModel 的 forward 方法,重写了 __call__
特殊方法。
尽管前向传递的配方需要在此函数内定义,但之后应该调用Module
实例而不是这个,因为前者负责运行预处理和后处理步骤,而后者会默默地忽略它们。