端到端:离线批量推理#
离线批量推理是在一组固定输入数据上生成模型预测的过程。Ray Data 提供了高效且可扩展的批量推理解决方案,为深度学习应用提供了更快的执行速度和成本效益。
关于为什么你应该使用 Ray Data 进行离线批量推理,以及它与替代方案的比较,请参阅 Ray Data 概述。
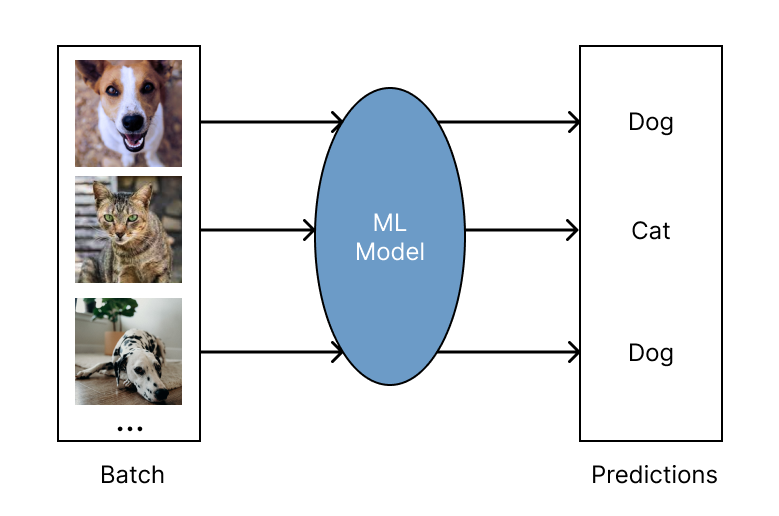
快速入门#
首先,安装 Ray Data:
pip install -U "ray[data]"
使用 Ray Data 进行离线推理涉及四个基本步骤:
步骤 1: 将您的数据加载到 Ray 数据集中。Ray 数据支持许多不同的数据源和格式。更多详情,请参阅 加载数据。
步骤 2: 定义一个 Python 类来加载预训练模型。
步骤 3: 通过调用
ds.map_batches()
使用预训练模型转换您的数据集。更多详情,请参阅 转换数据。
有关您使用案例的更深入示例,请参阅 批量推理示例。有关如何配置批量推理,请参阅 配置指南。
from typing import Dict
import numpy as np
import ray
# Step 1: Create a Ray Dataset from in-memory Numpy arrays.
# You can also create a Ray Dataset from many other sources and file
# formats.
ds = ray.data.from_numpy(np.asarray(["Complete this", "for me"]))
# Step 2: Define a Predictor class for inference.
# Use a class to initialize the model just once in `__init__`
# and re-use it for inference across multiple batches.
class HuggingFacePredictor:
def __init__(self):
from transformers import pipeline
# Initialize a pre-trained GPT2 Huggingface pipeline.
self.model = pipeline("text-generation", model="gpt2")
# Logic for inference on 1 batch of data.
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, list]:
# Get the predictions from the input batch.
predictions = self.model(list(batch["data"]), max_length=20, num_return_sequences=1)
# `predictions` is a list of length-one lists. For example:
# [[{'generated_text': 'output_1'}], ..., [{'generated_text': 'output_2'}]]
# Modify the output to get it into the following format instead:
# ['output_1', 'output_2']
batch["output"] = [sequences[0]["generated_text"] for sequences in predictions]
return batch
# Step 2: Map the Predictor over the Dataset to get predictions.
# Use 2 parallel actors for inference. Each actor predicts on a
# different partition of data.
predictions = ds.map_batches(HuggingFacePredictor, concurrency=2)
# Step 3: Show one prediction output.
predictions.show(limit=1)
{'data': 'Complete this', 'output': 'Complete this information or purchase any item from this site.\n\nAll purchases are final and non-'}
from typing import Dict
import numpy as np
import torch
import torch.nn as nn
import ray
# Step 1: Create a Ray Dataset from in-memory Numpy arrays.
# You can also create a Ray Dataset from many other sources and file
# formats.
ds = ray.data.from_numpy(np.ones((1, 100)))
# Step 2: Define a Predictor class for inference.
# Use a class to initialize the model just once in `__init__`
# and re-use it for inference across multiple batches.
class TorchPredictor:
def __init__(self):
# Load a dummy neural network.
# Set `self.model` to your pre-trained PyTorch model.
self.model = nn.Sequential(
nn.Linear(in_features=100, out_features=1),
nn.Sigmoid(),
)
self.model.eval()
# Logic for inference on 1 batch of data.
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, np.ndarray]:
tensor = torch.as_tensor(batch["data"], dtype=torch.float32)
with torch.inference_mode():
# Get the predictions from the input batch.
return {"output": self.model(tensor).numpy()}
# Step 2: Map the Predictor over the Dataset to get predictions.
# Use 2 parallel actors for inference. Each actor predicts on a
# different partition of data.
predictions = ds.map_batches(TorchPredictor, concurrency=2)
# Step 3: Show one prediction output.
predictions.show(limit=1)
{'output': array([0.5590901], dtype=float32)}
from typing import Dict
import numpy as np
import ray
# Step 1: Create a Ray Dataset from in-memory Numpy arrays.
# You can also create a Ray Dataset from many other sources and file
# formats.
ds = ray.data.from_numpy(np.ones((1, 100)))
# Step 2: Define a Predictor class for inference.
# Use a class to initialize the model just once in `__init__`
# and re-use it for inference across multiple batches.
class TFPredictor:
def __init__(self):
from tensorflow import keras
# Load a dummy neural network.
# Set `self.model` to your pre-trained Keras model.
input_layer = keras.Input(shape=(100,))
output_layer = keras.layers.Dense(1, activation="sigmoid")
self.model = keras.Sequential([input_layer, output_layer])
# Logic for inference on 1 batch of data.
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, np.ndarray]:
# Get the predictions from the input batch.
return {"output": self.model(batch["data"]).numpy()}
# Step 2: Map the Predictor over the Dataset to get predictions.
# Use 2 parallel actors for inference. Each actor predicts on a
# different partition of data.
predictions = ds.map_batches(TFPredictor, concurrency=2)
# Step 3: Show one prediction output.
predictions.show(limit=1)
{'output': array([0.625576], dtype=float32)}
配置与故障排除#
使用GPU进行推理#
要在推理中使用GPU,请对您的代码进行以下更改:
更新类实现以在GPU和CPU之间移动模型和数据。
在
ds.map_batches()
调用中指定num_gpus=1
,以指示每个执行者应使用1个GPU。指定一个
batch_size
用于推理。有关如何配置批量大小的更多详细信息,请参阅 配置批量大小。
其余部分与 快速开始 相同。
from typing import Dict
import numpy as np
import ray
ds = ray.data.from_numpy(np.asarray(["Complete this", "for me"]))
class HuggingFacePredictor:
def __init__(self):
from transformers import pipeline
# Set "cuda:0" as the device so the Huggingface pipeline uses GPU.
self.model = pipeline("text-generation", model="gpt2", device="cuda:0")
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, list]:
predictions = self.model(list(batch["data"]), max_length=20, num_return_sequences=1)
batch["output"] = [sequences[0]["generated_text"] for sequences in predictions]
return batch
# Use 2 actors, each actor using 1 GPU. 2 GPUs total.
predictions = ds.map_batches(
HuggingFacePredictor,
num_gpus=1,
# Specify the batch size for inference.
# Increase this for larger datasets.
batch_size=1,
# Set the concurrency to the number of GPUs in your cluster.
concurrency=2,
)
predictions.show(limit=1)
{'data': 'Complete this', 'output': 'Complete this poll. Which one do you think holds the most promise for you?\n\nThank you'}
from typing import Dict
import numpy as np
import torch
import torch.nn as nn
import ray
ds = ray.data.from_numpy(np.ones((1, 100)))
class TorchPredictor:
def __init__(self):
# Move the neural network to GPU device by specifying "cuda".
self.model = nn.Sequential(
nn.Linear(in_features=100, out_features=1),
nn.Sigmoid(),
).cuda()
self.model.eval()
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, np.ndarray]:
# Move the input batch to GPU device by specifying "cuda".
tensor = torch.as_tensor(batch["data"], dtype=torch.float32, device="cuda")
with torch.inference_mode():
# Move the prediction output back to CPU before returning.
return {"output": self.model(tensor).cpu().numpy()}
# Use 2 actors, each actor using 1 GPU. 2 GPUs total.
predictions = ds.map_batches(
TorchPredictor,
num_gpus=1,
# Specify the batch size for inference.
# Increase this for larger datasets.
batch_size=1,
# Set the concurrency to the number of GPUs in your cluster.
concurrency=2,
)
predictions.show(limit=1)
{'output': array([0.5590901], dtype=float32)}
from typing import Dict
import numpy as np
import ray
ds = ray.data.from_numpy(np.ones((1, 100)))
class TFPredictor:
def __init__(self):
import tensorflow as tf
from tensorflow import keras
# Move the neural network to GPU by specifying the GPU device.
with tf.device("GPU:0"):
input_layer = keras.Input(shape=(100,))
output_layer = keras.layers.Dense(1, activation="sigmoid")
self.model = keras.Sequential([input_layer, output_layer])
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, np.ndarray]:
import tensorflow as tf
# Move the input batch to GPU by specifying GPU device.
with tf.device("GPU:0"):
return {"output": self.model(batch["data"]).numpy()}
# Use 2 actors, each actor using 1 GPU. 2 GPUs total.
predictions = ds.map_batches(
TFPredictor,
num_gpus=1,
# Specify the batch size for inference.
# Increase this for larger datasets.
batch_size=1,
# Set the concurrency to the number of GPUs in your cluster.
concurrency=2,
)
predictions.show(limit=1)
{'output': array([0.625576], dtype=float32)}
配置批处理大小#
通过为 ds.map_batches()
设置 batch_size
参数,配置传递给 __call__
的输入批次的大小。
增加批次大小会导致更快的执行,因为推理是一个矢量化操作。对于GPU推理,增加批次大小会增加GPU利用率。将批次大小设置为尽可能大,而不会耗尽内存。如果遇到内存不足错误,减小 batch_size
可能会有所帮助。
import numpy as np
import ray
ds = ray.data.from_numpy(np.ones((10, 100)))
def assert_batch(batch: Dict[str, np.ndarray]):
assert len(batch) == 2
return batch
# Specify that each input batch should be of size 2.
ds.map_batches(assert_batch, batch_size=2)
小心
默认的 batch_size
为 4096
可能对于行数较大的数据集来说太大(例如,包含许多列的表格或大量大图像的集合)。
处理 GPU 内存不足的失败#
如果你遇到 CUDA 内存不足的问题,你的批处理大小可能太大。请按照 这些步骤 减少批处理大小。如果你的批处理大小已经设置为 1,那么请使用更小的模型或内存更大的 GPU 设备。
对于使用大型模型的高级用户,您可以使用模型并行性将模型分片到多个GPU上。
优化昂贵的CPU预处理#
如果你的工作负载涉及昂贵的CPU预处理以及模型推理,你可以通过将预处理和推理逻辑分离到不同的操作中来优化吞吐量。这种分离使得批处理 \(N\) 的推理可以与批处理 \(N+1\) 的预处理同时执行。
有关在单独的 map
调用中进行预处理的示例,请参见 使用 PyTorch ResNet18 进行图像分类批量推理。
处理CPU内存不足的故障#
如果你耗尽了 CPU 内存,你可能在同一个节点上运行了太多的模型副本。例如,如果一个模型在创建/运行时使用了 5 GB 的 RAM,而一台机器总共有 16 GB 的 RAM,那么同一时间最多只能运行三个这样的模型。每个任务/角色的默认资源分配为一个 CPU 可能会导致 Ray 在这种情况下出现 OutOfMemoryError
。
假设你的集群有4个节点,每个节点有16个CPU。为了限制每个节点最多3个这样的执行者,你可以覆盖CPU或内存:
from typing import Dict
import numpy as np
import ray
ds = ray.data.from_numpy(np.asarray(["Complete this", "for me"]))
class HuggingFacePredictor:
def __init__(self):
from transformers import pipeline
self.model = pipeline("text-generation", model="gpt2")
def __call__(self, batch: Dict[str, np.ndarray]) -> Dict[str, list]:
predictions = self.model(list(batch["data"]), max_length=20, num_return_sequences=1)
batch["output"] = [sequences[0]["generated_text"] for sequences in predictions]
return batch
predictions = ds.map_batches(
HuggingFacePredictor,
# Require 5 CPUs per actor (so at most 3 can fit per 16 CPU node).
num_cpus=5,
# 3 actors per node, with 4 nodes in the cluster means concurrency of 12.
concurrency=12,
)
predictions.show(limit=1)