什么是 Ray Core?#
Ray Core 提供了少量核心原语(即任务、角色、对象),用于构建和扩展分布式应用程序。下面我们将通过简单的示例,展示如何轻松地将您的函数和类转换为 Ray 任务和角色,以及如何使用 Ray 对象。
入门指南#
要开始使用,请通过 pip install -U ray
安装 Ray。有关更多安装选项,请参阅 安装 Ray。接下来的几节将介绍使用 Ray Core 的基础知识。
第一步是导入并初始化 Ray:
import ray
ray.init()
备注
在Ray的最新版本(>=1.5)中,首次使用Ray远程API时会自动调用 ray.init()
。
运行任务#
Ray 允许你在集群中将函数作为远程任务运行。为此,你可以使用 @ray.remote
装饰你的函数,以声明你希望远程运行此函数。然后,你可以使用 .remote()
调用该函数,而不是正常调用它。这个远程调用返回一个未来,即所谓的 Ray 对象引用,你可以使用 ray.get
获取它:
# Define the square task.
@ray.remote
def square(x):
return x * x
# Launch four parallel square tasks.
futures = [square.remote(i) for i in range(4)]
# Retrieve results.
print(ray.get(futures))
# -> [0, 1, 4, 9]
调用一个演员#
Ray 提供了 actors 来允许你在多个 actor 实例之间并行化计算。当你实例化一个 Ray actor 类时,Ray 将在集群中启动该类的远程实例。这个 actor 可以执行远程方法调用并维护其自己的内部状态:
# Define the Counter actor.
@ray.remote
class Counter:
def __init__(self):
self.i = 0
def get(self):
return self.i
def incr(self, value):
self.i += value
# Create a Counter actor.
c = Counter.remote()
# Submit calls to the actor. These calls run asynchronously but in
# submission order on the remote actor process.
for _ in range(10):
c.incr.remote(1)
# Retrieve final actor state.
print(ray.get(c.get.remote()))
# -> 10
上述内容涵盖了非常基本的参与者使用方法。如需更深入的示例,包括同时使用任务和参与者,请查看 蒙特卡罗法估计 π。
传递一个对象#
如上所述,Ray 将其任务和 actor 调用结果存储在其 分布式对象存储 中,返回 对象引用 ,这些引用可以在以后检索。对象引用也可以通过 ray.put
显式创建,并且对象引用可以作为参数值的替代传递给任务:
import numpy as np
# Define a task that sums the values in a matrix.
@ray.remote
def sum_matrix(matrix):
return np.sum(matrix)
# Call the task with a literal argument value.
print(ray.get(sum_matrix.remote(np.ones((100, 100)))))
# -> 10000.0
# Put a large array into the object store.
matrix_ref = ray.put(np.ones((1000, 1000)))
# Call the task with the object reference as an argument.
print(ray.get(sum_matrix.remote(matrix_ref)))
# -> 1000000.0
下一步#
小技巧
要检查您的应用程序的运行情况,您可以使用 Ray 仪表盘。
Ray 的核心原语很简单,但可以组合在一起表达几乎任何类型的分布式计算。通过以下用户指南了解更多关于 Ray 的 关键概念:
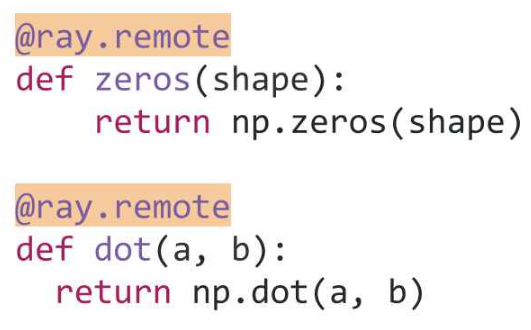
使用远程函数 (Tasks)
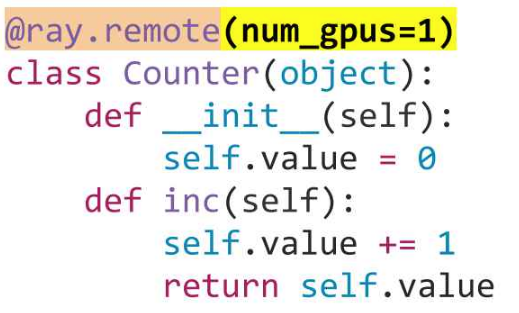
使用远程类(Actor)
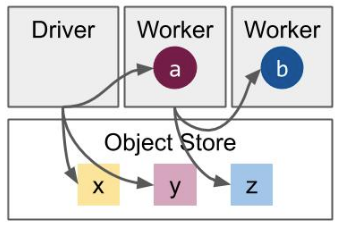
使用 Ray 对象