Note
Go to the end to download the full example code. or to run this example in your browser via Binder
瑞士卷和瑞士洞降维#
本笔记本旨在比较两种流行的非线性降维技术,T-分布随机邻嵌入(t-SNE)和局部线性嵌入(LLE),在经典的瑞士卷数据集上的表现。然后,我们将探讨它们如何处理数据中添加一个洞的情况。
Swiss Roll#
我们首先生成瑞士卷数据集。
import matplotlib.pyplot as plt
from sklearn import datasets, manifold
sr_points, sr_color = datasets.make_swiss_roll(n_samples=1500, random_state=0)
现在,让我们来看一下我们的数据:
fig = plt.figure(figsize=(8, 6))
ax = fig.add_subplot(111, projection="3d")
fig.add_axes(ax)
ax.scatter(
sr_points[:, 0], sr_points[:, 1], sr_points[:, 2], c=sr_color, s=50, alpha=0.8
)
ax.set_title("Swiss Roll in Ambient Space")
ax.view_init(azim=-66, elev=12)
_ = ax.text2D(0.8, 0.05, s="n_samples=1500", transform=ax.transAxes)
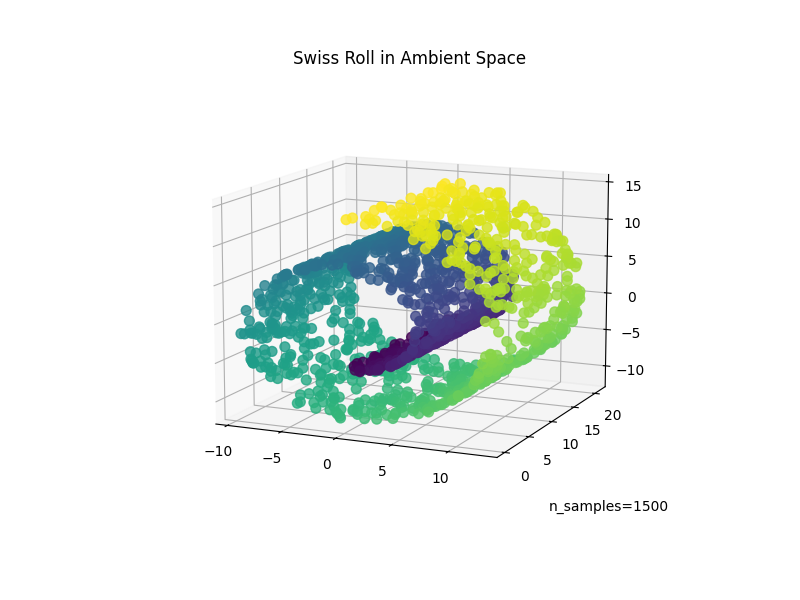
计算LLE和t-SNE嵌入,我们发现LLE似乎能够非常有效地展开瑞士卷。另一方面,t-SNE能够保留数据的一般结构,但不能很好地表示我们原始数据的连续性。相反,它似乎不必要地将一些点聚集在一起。
sr_lle, sr_err = manifold.locally_linear_embedding(
sr_points, n_neighbors=12, n_components=2
)
sr_tsne = manifold.TSNE(n_components=2, perplexity=40, random_state=0).fit_transform(
sr_points
)
fig, axs = plt.subplots(figsize=(8, 8), nrows=2)
axs[0].scatter(sr_lle[:, 0], sr_lle[:, 1], c=sr_color)
axs[0].set_title("LLE Embedding of Swiss Roll")
axs[1].scatter(sr_tsne[:, 0], sr_tsne[:, 1], c=sr_color)
_ = axs[1].set_title("t-SNE Embedding of Swiss Roll")
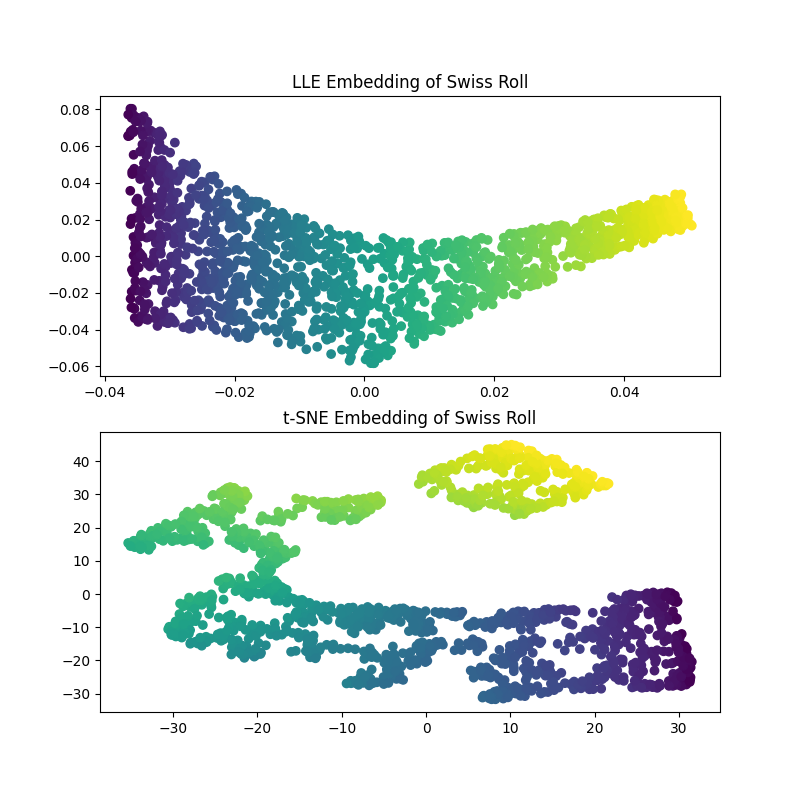
LLE 似乎正在拉伸瑞士卷中心(紫色)的点。然而,我们观察到这只是数据生成方式的副产品。瑞士卷中心附近的点密度较高,这最终影响了 LLE 在低维度上重建数据的方式。
Swiss-Hole#
现在让我们看看这两种算法如何处理我们在数据中添加一个空洞。首先,我们生成瑞士卷空洞数据集并绘制它:
sh_points, sh_color = datasets.make_swiss_roll(
n_samples=1500, hole=True, random_state=0
)
fig = plt.figure(figsize=(8, 6))
ax = fig.add_subplot(111, projection="3d")
fig.add_axes(ax)
ax.scatter(
sh_points[:, 0], sh_points[:, 1], sh_points[:, 2], c=sh_color, s=50, alpha=0.8
)
ax.set_title("Swiss-Hole in Ambient Space")
ax.view_init(azim=-66, elev=12)
_ = ax.text2D(0.8, 0.05, s="n_samples=1500", transform=ax.transAxes)
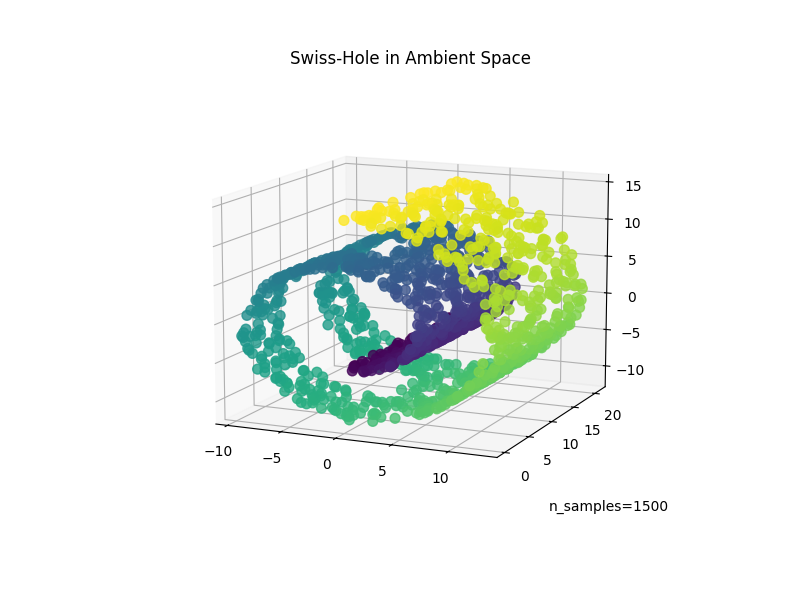
计算LLE和t-SNE嵌入,我们得到与瑞士卷相似的结果。LLE非常有效地展开了数据,甚至保留了孔洞。t-SNE再次将部分点聚集在一起,但我们注意到它保留了原始数据的一般拓扑结构。
sh_lle, sh_err = manifold.locally_linear_embedding(
sh_points, n_neighbors=12, n_components=2
)
sh_tsne = manifold.TSNE(
n_components=2, perplexity=40, init="random", random_state=0
).fit_transform(sh_points)
fig, axs = plt.subplots(figsize=(8, 8), nrows=2)
axs[0].scatter(sh_lle[:, 0], sh_lle[:, 1], c=sh_color)
axs[0].set_title("LLE Embedding of Swiss-Hole")
axs[1].scatter(sh_tsne[:, 0], sh_tsne[:, 1], c=sh_color)
_ = axs[1].set_title("t-SNE Embedding of Swiss-Hole")
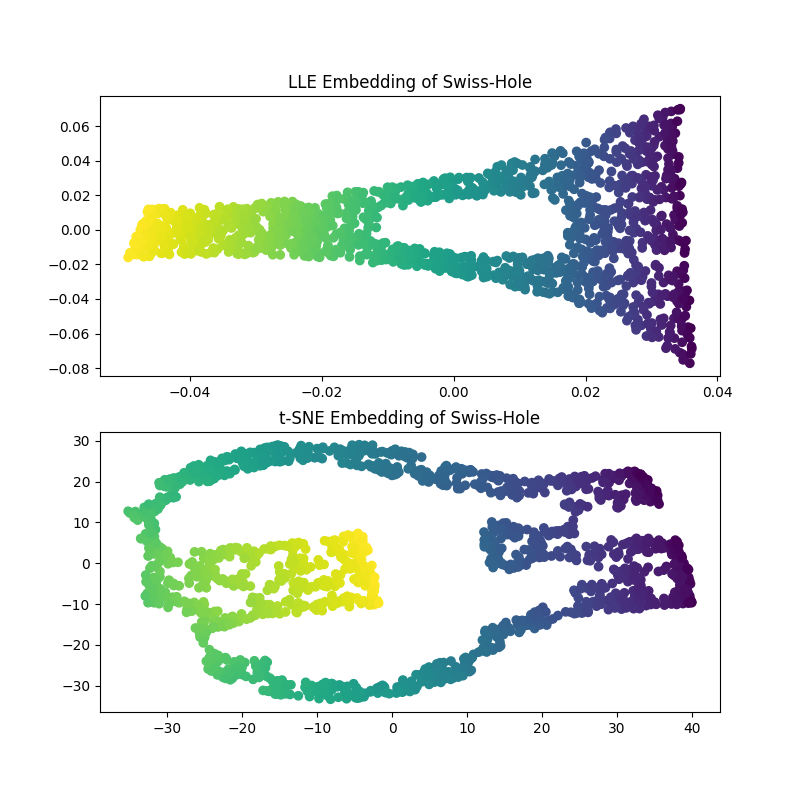
结论#
我们注意到,t-SNE 通过测试更多的参数组合可以受益。通过更好地调整这些参数,可能会获得更好的结果。
我们观察到,如“手写数字上的流形学习”示例所示,t-SNE 通常在真实世界数据上比 LLE 表现更好。
Total running time of the script: (0 minutes 28.131 seconds)
Related examples