Note
Go to the end to download the full example code. or to run this example in your browser via Binder
使用部分依赖的高级绘图#
PartialDependenceDisplay
对象可以用于绘图,而无需重新计算部分依赖。在这个示例中,我们展示了如何绘制部分依赖图以及如何使用可视化 API 快速自定义图表。
Note
另请参见 ROC 曲线与可视化 API
import matplotlib.pyplot as plt
import pandas as pd
from sklearn.datasets import load_diabetes
from sklearn.inspection import PartialDependenceDisplay
from sklearn.neural_network import MLPRegressor
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.tree import DecisionTreeRegressor
在糖尿病数据集上训练模型#
首先,我们在糖尿病数据集上训练一个决策树和一个多层感知器。
diabetes = load_diabetes()
X = pd.DataFrame(diabetes.data, columns=diabetes.feature_names)
y = diabetes.target
tree = DecisionTreeRegressor()
mlp = make_pipeline(
StandardScaler(),
MLPRegressor(hidden_layer_sizes=(100, 100), tol=1e-2, max_iter=500, random_state=0),
)
tree.fit(X, y)
mlp.fit(X, y)
绘制两个特征的部分依赖图#
我们为决策树的特征“age”和“bmi”(身体质量指数)绘制部分依赖曲线。对于两个特征,from_estimator
期望绘制两条曲线。这里,绘图函数使用 ax
定义的空间放置了一个包含两个图的网格。
fig, ax = plt.subplots(figsize=(12, 6))
ax.set_title("Decision Tree")
tree_disp = PartialDependenceDisplay.from_estimator(tree, X, ["age", "bmi"], ax=ax)
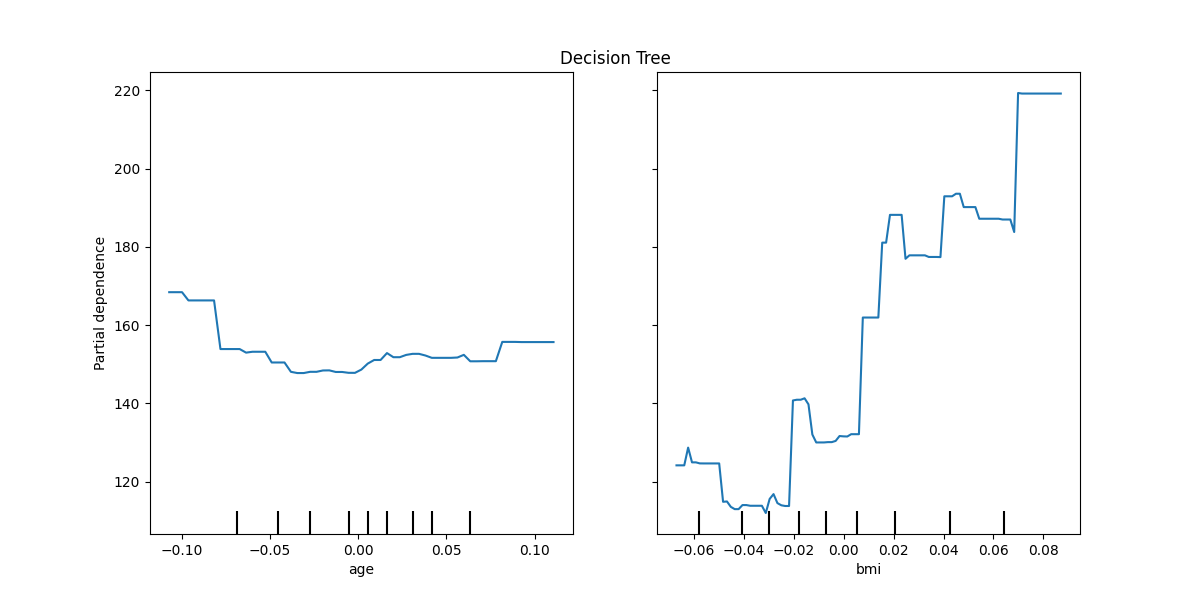
可以为多层感知机绘制部分依赖曲线。在这种情况下, line_kw
被传递给 from_estimator
以更改曲线的颜色。
fig, ax = plt.subplots(figsize=(12, 6))
ax.set_title("Multi-layer Perceptron")
mlp_disp = PartialDependenceDisplay.from_estimator(
mlp, X, ["age", "bmi"], ax=ax, line_kw={"color": "red"}
)
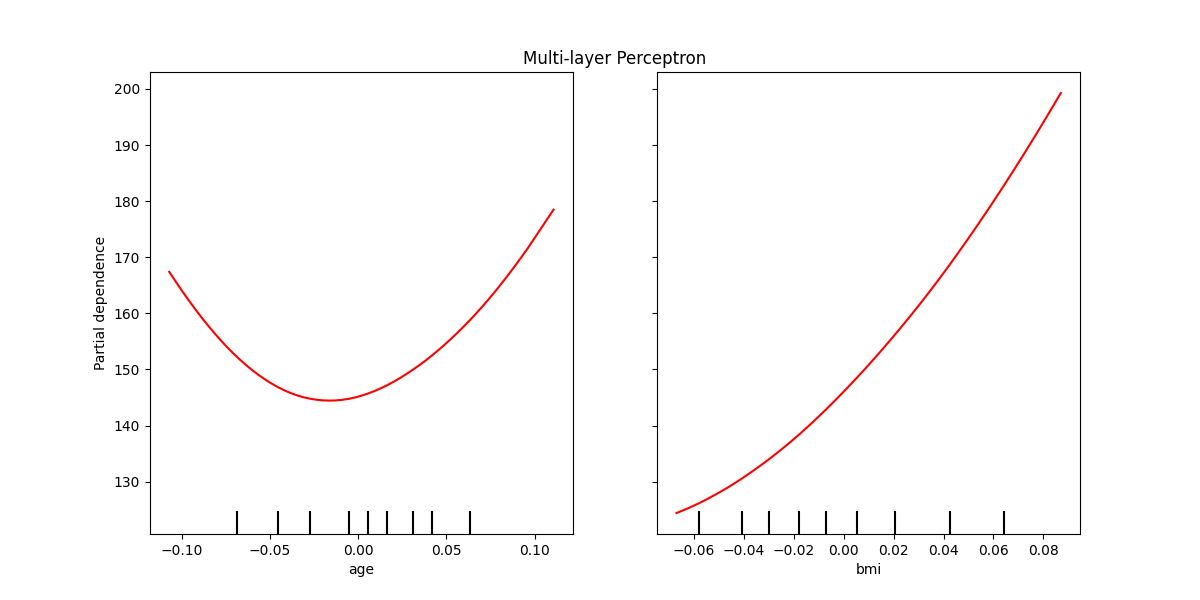
绘制两个模型的部分依赖图#
tree_disp
和 mlp_disp
PartialDependenceDisplay
对象包含了重新创建部分依赖曲线所需的所有计算信息。这意味着我们可以轻松创建额外的图表,而无需重新计算曲线。
一种绘制曲线的方法是将它们放在同一个图中,每个模型的曲线位于每一行。首先,我们创建一个包含两行一列的图形,并在其中放置两个坐标轴。这两个坐标轴将传递给 tree_disp
和 mlp_disp
的 plot
函数。给定的坐标轴将被绘图函数用来绘制部分依赖图。最终的图将决策树的部分依赖曲线放在第一行,多层感知器的部分依赖曲线放在第二行。
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(10, 10))
tree_disp.plot(ax=ax1)
ax1.set_title("Decision Tree")
mlp_disp.plot(ax=ax2, line_kw={"color": "red"})
ax2.set_title("Multi-layer Perceptron")
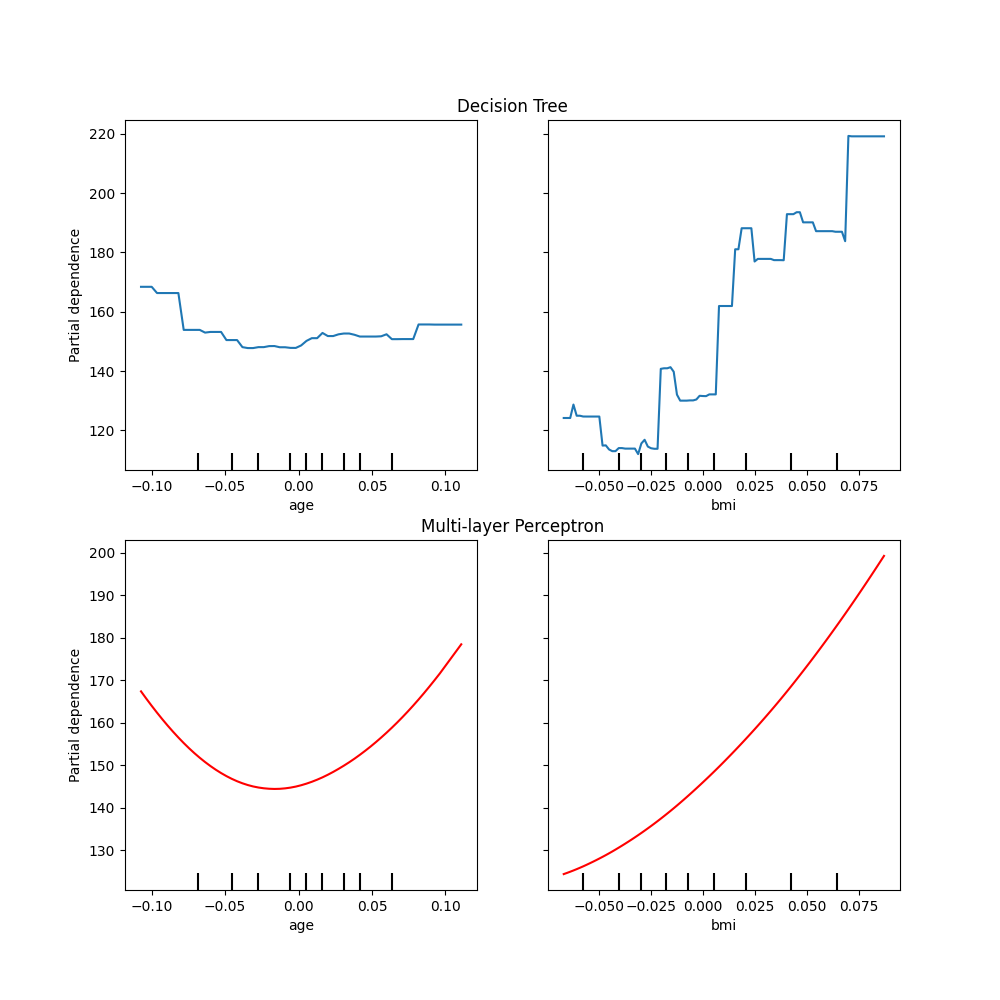
Text(0.5, 1.0, 'Multi-layer Perceptron')
另一种比较曲线的方法是将它们叠加在一起绘制。在这里,我们创建一个包含一行两列的图形。将坐标轴作为列表传递给 plot
函数,该函数将在相同的坐标轴上绘制每个模型的部分依赖曲线。坐标轴列表的长度必须等于绘制的图的数量。
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 6))
tree_disp.plot(ax=[ax1, ax2], line_kw={"label": "Decision Tree"})
mlp_disp.plot(
ax=[ax1, ax2], line_kw={"label": "Multi-layer Perceptron", "color": "red"}
)
ax1.legend()
ax2.legend()
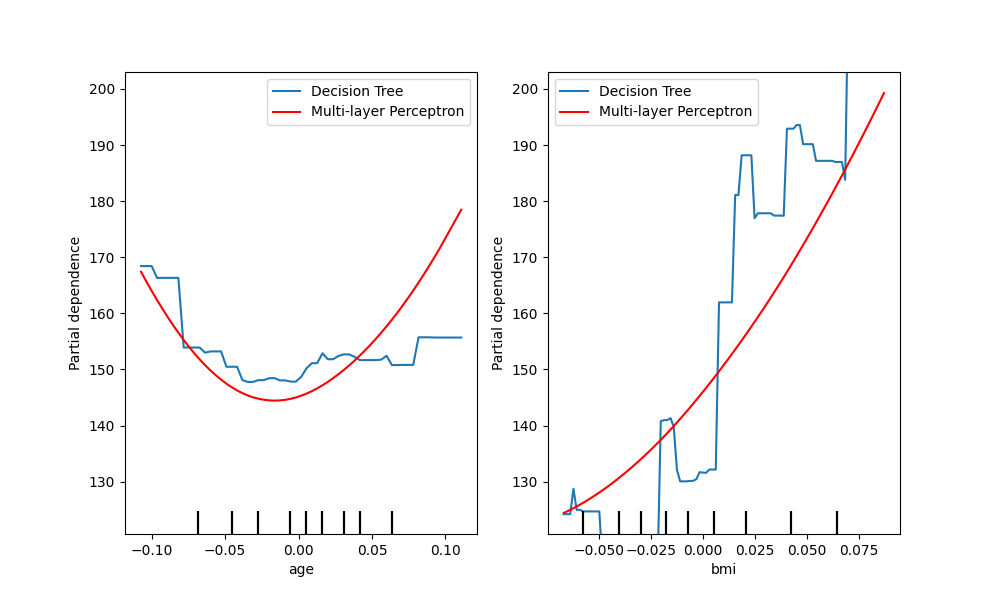
<matplotlib.legend.Legend object at 0xffff86fd0c10>
tree_disp.axes_
是一个 numpy 数组,包含用于绘制部分依赖图的坐标轴。可以将其传递给 mlp_disp
,以实现将图形叠加绘制的效果。此外, mlp_disp.figure_
存储了图形对象,这允许在调用 plot
之后调整图形的大小。在这种情况下, tree_disp.axes_
有两个维度,因此 plot
只会在最左边的图上显示 y 轴标签和 y 轴刻度。
tree_disp.plot(line_kw={"label": "Decision Tree"})
mlp_disp.plot(
line_kw={"label": "Multi-layer Perceptron", "color": "red"}, ax=tree_disp.axes_
)
tree_disp.figure_.set_size_inches(10, 6)
tree_disp.axes_[0, 0].legend()
tree_disp.axes_[0, 1].legend()
plt.show()
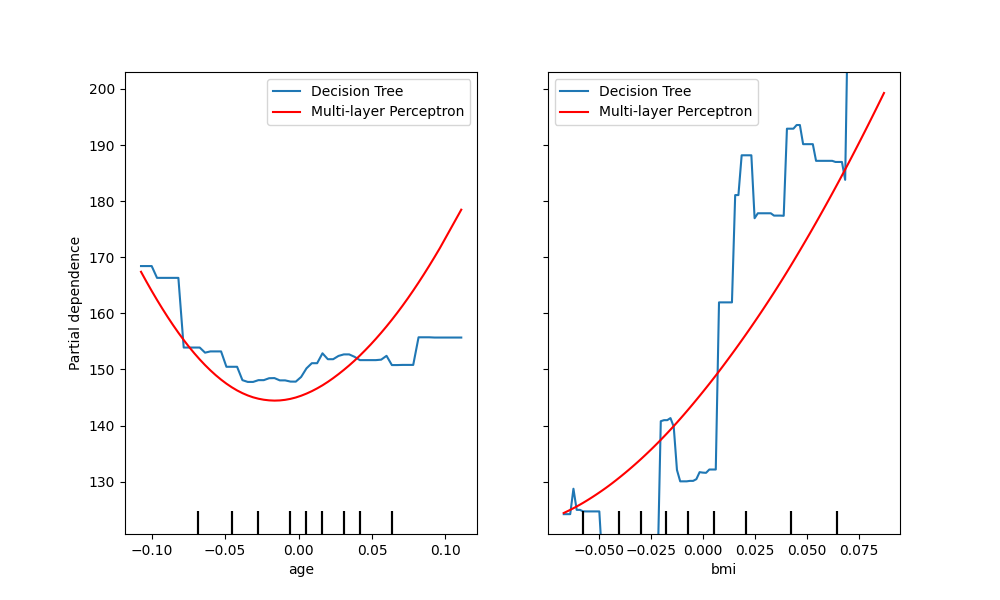
绘制单个特征的部分依赖图#
在这里,我们在同一坐标轴上绘制单个特征“年龄”的部分依赖曲线。在这种情况下, tree_disp.axes_
被传递到第二个绘图函数中。
tree_disp = PartialDependenceDisplay.from_estimator(tree, X, ["age"])
mlp_disp = PartialDependenceDisplay.from_estimator(
mlp, X, ["age"], ax=tree_disp.axes_, line_kw={"color": "red"}
)
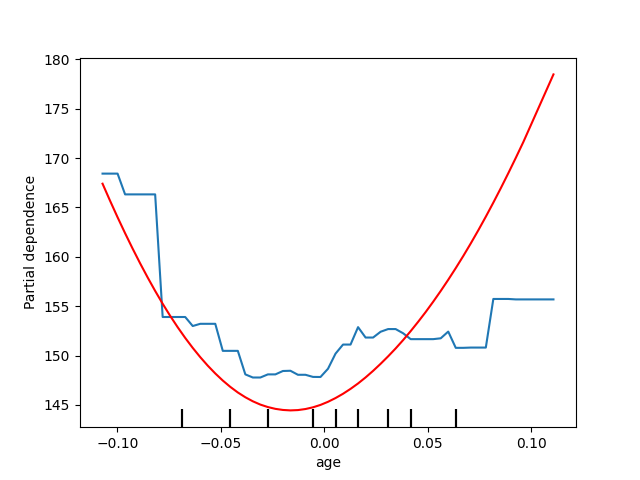
Total running time of the script: (0 minutes 1.289 seconds)
Related examples